**Principles of Computer Graphics**
**Reed College CSCI 385 Fall 2024**
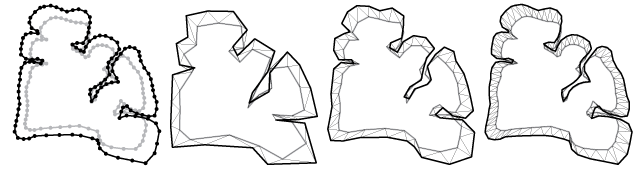
......................... . . . . . . . . . . . . .
This course is an introduction to the mathematical modeling used for
computer graphics applications. Topics include coordinate systems,
vector and affine spaces, 2-D and 3-D transforms, projection, curves
and surfaces, and animation. We work to produce photorealistic 2-D
renderings of 3-D virtual scenes. To do so we use physical modeling
and simulation to mimic natural phenomena. To produce the images
quickly enough for interaction, we use numeric approximation
techniques and graphics hardware, and also geometric algorithms and
data structures.
There will be several programming projects to work with and showcase
these principles and methods. Each project will be an interactive web
page demonstrating your work. We will code in Javascript, using WebGL
libraries to take advantage of the graphics hardware on your computer.
**Prerequisites:** CSCI 121 and MATH 201.
**Meets:** 10:30-11:50 TuTh in TRL 150.
**Instructor:** Jim Fix
**Office:** Library 314
Jim's office hours are posted [here](http://jimfix.github.io/teaching.html).
# Schedule
To be laid out as things unfold.
**Week 1**: overview and preliminaries
. course overview: [slides A](lectures/CG-Lec01-1a.pdf) . [slides B](lectures/CG-Lec01-1b.pdf) . [syllabus](CG-f24-syllabus.pdf) . [Stanford bunny](demos/viewer/bunny.html) . [Utah teapot](demos/viewer/teapot.html)
. Javascript and WebGL; [slides](lectures/CG-Lec01-2.pdf) . [js](js/primer.html) . [samples](js/CG-Lec01-2-js.zip) . [**demo**](demos/webgl/triangle.html)
**Project 0** [**try it**](projects/project0/try-it.html) *due: 9/10*
**Week 2**: geometry, coloring, and framing
. triangle demo, revisited; legacy OpenGL: [**slides**](lectures/CG-Lec02-1.pdf) . [**triangle OpenGL**](demos/triangle-opengl/triangle-opengl.html)
. RGB space; transformation stack:
. [**slides**](lectures/CG-Lec02-2.pdf)
. [**a pretty scene**](demos/scene/scene.html)
. [**hand-waving**](demos/hand-waving/hand-waving.html)
. [**xform F**](demos/fffft/fffft.html)
**Project 1** [**object showcase**](projects/project1/showcase.html) *due: 9/19*
**Week 3**: points and vectors
. vector and affine spaces; homogeneous coordinates: [**slides**](lectures/CG-Lec03-1and2.pdf)
. [DeRose et al. paper](https://cs.uwaterloo.ca/research/tr/1997/15/CS-97-15.pdf)
**Project 2** [**make a scene**](projects/project2/scene.html) *due: 10/1*
**Week 4**: Euclidean 3-space; Projection
. inner product, angles, and distance; cross product; rotation: [**slides**](lectures/CG-Lec04-1.pdf)
. viewing and projection; perspective: [**slides**](lectures/CG-Lec04-2.pdf)
**Week 5**: Interpolation; Curves
. projection (cont'd); Lagrange interpolation: [**slides**](lectures/CG-Lec05-1.pdf)
. Bezier curves: [**demo**](demos/bezier/bezier2.html) [**demo**](demos/bezier/bezier3.html) [**slides**](lectures/CG-Lec05-2.pdf)
**Project 3** [**walk thru it**](projects/project3/walk-thru-it.html) *due: 10/17*
**Week 6**: Project 3 write-up; Curves (cont'd)
. solutions to the written part of Project 3 are [**here**](projects/project3/walk-thru-it-solution.pdf)
. B-spline curves: [**slides**](lectures/CG-Lec06-1and2.pdf)
**Week 7**: B-splines (cont'd); Quaternions
. B-spline derivation; subdivision: [**slides**](lectures/CG-Lec07-1.pdf)
. rotation interpolation; quaternions: [**slides**](lectures/CG-Lec07-2.pdf) [**slerp demo**](demos/slerp/slerp.html)
**Week 8**: rendering; illumination; shading
. the graphics pipeline and rasterization: [**slides**](lectures/CG-Lec08-1.pdf)
**Project 4** [**the swerve**](projects/project4/swerve-thru-it.html) *due: 11/7*
. Phong reflection and shading: [**slides**](lectures/CG-Lec08-2.pdf)
**Week 9**: ray casting and tracing in GLSL
. ray casting and tracing: [**slides**](lectures/CG-Lec09.pdf) [**demo**](demos/ray/ray-cast/ray-cast.html)
**Project 5** [**Bezier funhouse**](projects/project5/bezier-funhouse.html) *due: 11/21*
. work in-progress: [**refraction demo**](demos/ray/ray-cast-transmit/ray-cast.html)
**Week 10**: surfaces
. implicit surfaces, marching cubes, meshes: [**slides**](lectures/CG-Lec10-1.pdf)
. Bezier and B-spline patches; subdivision surfaces: [**slides**](lectures/CG-Lec10-2.pdf)
**Week 11**: physical simulation
. particle systems, Euler's method: [**slides**](lectures/CG-Lec11-1.pdf)
. Reynold's boids; improving upon Euler: [**slides**](lectures/CG-Lec11-2.pdf)
**Project 6** [**cycle subdivide**](projects/project6/cycle-subdivide.html) *due: 12/3*
**Week 12**: thanksgiving
**Project 7** [**one sheet**](projects/project7/sheet-to-the-wind.html) *due: 12/19*
**Week 13**: more physical simulation
. fluids: [**slides**](lectures/CG-Lec13-1.pdf) (see links)
. animation principles; spacetime constraints: [**slides**](lectures/CG-Lec13-2.pdf)
......................... . . . . . . . . . . . . . . . .
# Topics
The topics below are what we work to cover in this course, listed roughly
in the order we'll cover them. This info will get fleshed out as the
semester progresses into a week-by-week collection of lecture materials and
project links just above.
**WebGL**

. introduction to Javascript,
. interactive web pages.
. WebGL and OpenGL for graphics rendering.
**Geometry I**

. 3-D surface representation.
. linear and affine spaces.
. interpolation; barycentric coordinates.
**Geometry II**

. viewing and model transformations
. matrix representation.
. homogenous coordinates.
. coordinate frames.
. projection.
**Curves**

. position interpolation.
. Bezier and B-spline curves.
. subdivision curves.
**Rendering I**

. direct illumination, ray casting, and z-buffering.
. shading and lighting models.
. vertex and fragment shaders in GLSL.
. the graphics (hardware) pipeline.
**Surfaces**

. mesh data structures.
. Bezier and B-spline patches.
. subdivision surfaces.
**Rendering II**

. recursive ray tracing.
. reflection, refraction, shadows.
. global illumination.
**Animation**

. classic animation; keyframing; animation principles.
. quaternions for interpolating orientation.
. particle systems; masses & springs.
. numeric integration for modeling motion.
......................... . . . . . . . . . . . . . . . .
# Assignments
There will be six (or seven, time permitting) programming projects, each covering some portion of the topics above. They are of varying scope and challenge. Some of these projects have a written component that serves either as a warm-up or as a chance to make the calculations needed to complete the project. All of the projects can be completed individually but, for a few, I will allow you to work with a partner and hand in a single project. In that case, the work must truly be collaborative.
The current plan for the suite of projects is as follows:
* **Program 1: *the object showcase.* ** Geometric modeling of surfaces, rendered with an OpenGL-like Javascript library in WebGL.
* **Program 2: *make a scene.* ** Lay out 2D and 3D scenes, one recursively constructed, using transformations.
* **Program 3: *walk thru it.* ** Produce a flip-book of a camera's path through a scene with ray casting and hidden line removal.
* **Program 4: *the swerve.* ** Make the Program 3 path smoothly vary, both in position and in orientation.
* **Program 5: *the Bezier funhouse.* ** A hardware-level ray-trace of a scene with curved mirrors and shadows.
* **Program 6: *cycle subdivide.* ** Make a Tron-like game that is played on a smooth subdivision surface.
* **Program 7: *one sheet to the wind.* ** Model fabric that is blown by a breeze.
There is a specific variety of applied mathematics we are
learning this semester, and that perhaps is another main goal of the
course--- concretely and directly using several mathematical
techniques for modeling and simulation. You'll hopefully work out a
lot on paper before you code up your ideas. I strongly encourage
working this way.
**Bugs**
Programming that performs graphical modeling and output
is the main goal of the course, and it is a peculiar variety of coding.
Bugs are literally made apparent--- you *see* your mistakes. Oftentimes
the code runs just fine, but the output looks wrong or off. You'll develop
a wide set of skills for anticipating bugs, and the bugs can be numeric
mistakes as well as the logical, logistical, and other traditional coding
bugs that you normally encounter writing code.
This sometimes makes the programming fun. I encourage you to save any
["happy accidents"](https://www.youtube.com/watch?v=_Tq5vXk0wTk) that
result from buggy code. You can collect a
[blooper reel](https://dl.acm.org/doi/abs/10.1145/77276.77289)
of the interesting artifacts of mistakes throughout the semester.
**Clarity, Style, and Documentation**
All the work you turn in is evaluated not only for its correctness, but also in how it is presented. Clearly document your code. Write it so that it’s easy for others to understand. Include separate documentation of how it works and what it does. Tell me what works and what’s broken. For this course I will often give more credit for something that is well-documented even if it has a known bug. Oftentimes there is one slight problem that is easy to fix. You telling me that you are aware of the problem is better than me finding it on my own and wondering whether or not you were aware of it.
Screenshots are particularly helpful in this course for communicating how things function. If there are any quirks or bugs, and also any extra things you’ve done beyond the spec, a picture of it is worth a lot.
**Academic Integrity**
For work done solo, the work you turn in must be yours and yours alone. For partnering, it must only be yours and your partner’s work. We take this very seriously and will report any violations of this policy. In particular, you cannot copy others’ code or share your code with others.
Discussing and working out your ideas and formulations together, though, is highly encouraged. We will often take time in class to do so, sharing our approaches to solve a computer graphics problem. You may also do that outside of the classroom, but you should do so judiciously. Taking someone’s idea or calculation is very different than working together and hashing out your different approaches. In cases where you have a close collaboration with someone (and it is not a formal partner for a project) the right thing to do is to tell us who you worked with, and how, when you turn in that work.
The same care should be taken with anything you find on-line, in texts, and in sources of code. Since there are no official readings for this work, I expect you to do some outside reading and exploring of the wide array of computer graphics resources available to you. In doing so, you cannot directly steal code or formulations that solve the key puzzles that are core to completing the work of an assignment.
If you are unsure about situations that might arise related to the above, in working with others or using outside resources, please consult with me. And, again, it is always right to cite someone or some resource you relied upon. You should always communicate where you got your ideas and how you completed any of your work.
......................... . . . . . . . . . . . . . . . .
# Outcomes
Students in this course will do the following:
* Review linear algebra in the context of computer graphics. This includes 4-D transformations of points and vectors represented with homogeneous coordinates.
* Learn the principles of the numeric modeling of curves and surfaces for geometric design, especially B-spline, Bezier, and subdivision curves and surfaces.
* Learn the standard hardware and software techniques for modeling color, light, and shading in complex 3-D scenes and rendering them from some viewpoint.
* Develop and debug interactive visual programs.
* Learn numeric techniques for interpolation, differentiation, and integration, particularly for physical modeling and animation.
* Write code that uses geometric data structures and algorithms.
* Review research developments in the field of computer graphics (e.g. activities of SIGGRAPH).
......................... . . . . . . . . . . . . . . . .
# Texts and References
There are several good computer graphics textbooks that all roughly
cover the same material. I've worked to make a complete list just
below here. Many vary simply because they include code from a
particular language and library, say OpenGL/C++ or WebGL/Javascript.
I've chosen to make three particular texts available in the bookstore
and in the library. They are not required, but obtaining at least one
of them is strongly recommended. Any of the three could be treated as
a textbook of the course, and they all cover the core material that we
will cover.
**Recommended**
. Marschner & Shirley, [*Fundamentals of Computer Graphics*](http://www.cs.cornell.edu/~srm/fcg4/),
[5th edition](https://www.routledge.com/Fundamentals-of-Computer-Graphics/Marschner-Shirley/p/book/9780367505035)
CRC Press, 2021.
. Angel & Shreiner, [*Interactive Computer Graphics*](https://www.pearson.com/us/higher-education/product/ANGEL-Interactive-Computer-Graphics-RENTAL-EDITION-8th-Edition/9780136681670.html),
8th edition (WebGL), Pearson, 2020.
. Gortler, [*Foundations of 3D Computer Graphics*](http://www.3dgraphicsfoundations.com/), MIT Press, 2012.
The last, Gortler, is the tersest, but I find the mathematics more in
line with what you might expect from Reed math (and physics)
courses. In a way, it lacks fluff and so is most succinct in relating
the ideas and the techniques. It's stronger with the preliminaries,
namely, in its coordinate-free approach to specifying scene geometry,
transformations of scene objects' geometry, and scene view
transformations. But Gortler is, at times, fairly cavalier in its
approach to the other topics. It is the cheapest, as a result.
The Angel & Shreiner text does a better job of covering the rest of
the standard graphics techniques. The latest two editions are written
with WebGL, the graphics library we use. The Marschner & Shirley text,
is maybe the best in between. It covers a lot more material than
Gortler, roughly the same as what's in Angel & Shreiner, but its
exposition of the mathematics feels more clean and direct than
Angel & Shreiner.
**Others**
. Blinn, [Jim Blinn's Corner: (various subtitles, three volumes](http://www.jimblinn.com/publications/).
. Buss, [3-D Computer Graphics](http://www.math.ucsd.edu/~sbuss/MathCG/index.html).
. Casselman, [Mathematical Illustrations](http://www.math.ubc.ca/~cass/graphics/manual/index.html).
. Cohen & Wallace, [Radiosity and Realistic Image Synthesis](http://www.amazon.com/Radiosity-Realistic-Synthesis-Kaufmann-Computer/dp/0121782700).
. DeRose, [3-D Computer Graphics: a Coordinate-Free Approach](https://graphics.pixar.com/people/derose/publications/GeometryBook/paper.pdf).
. Foley & van Dam, [Computer Graphics Principles and Practice](http://www.amazon.com/Computer-Graphics-Principles-Practice-Edition/dp/0321399528).
. Glassner, [An Introduction to Ray Tracing](http://www.glassner.com/portfolio/an-introduction-to-ray-tracing/).
. Glassner, [Principles of Digital Image Synthesis (volumes 1 and 2)](http://www.realtimerendering.com/Principles_of_Digital_Image_Synthesis_v1.0.1.pdf).
Foley & van Dam is the most renowned text. Its first edition was one
of the first of its kind. It was recently updated but the two older
versions are also worth looking at, especially to see in what ways
graphics technology has, and hasn't, changed. Of the remaining, I
found Peter Shirley's and Samuel Buss' to be the most focused on the
mathematical fundamentals of the field. (I should note that Peter
Shirley is an alum.)
**SIGGRAPH proceedings**
The best collection of materials on computer graphics, including the
most up-to-date write-ups of techniques, can be found in the research
paper proceedings of [the annual SIGGRAPH conference](https://s2024.siggraph.org/)
The 2024 conference's technical papers are [linked here](https://dl.acm.org/doi/proceedings/10.1145/3641519) through the ACM digital library. I highly
recommend browsing through several recent years, and also ones from
many years' past, to get a sense of what the computer graphics
research community works on. The papers are generally very readable
to anyone with a solid undergraduate math (and/or physics) background.
We will look at papers and tutorials from this conference throughout
the semester.
......................... . . . . . . . . . . . . . . . .
# Programming Resources
We will be writing code that relies on WebGL, WebGLUtil, GL-matrix and
other supporting Javascript libraries. These all rely on graphics
hardware and are built on top of OpenGL, a standard library (normally
invoked in C++) for communicating with GPUs to do fast hardware
rendering. In the last decade, all these GL-based language tools
rely on *vertex and fragment shaders* written in GLSL, a C-like
language for calculating on the GPU. There are a wealth of resources
on-line for these technologies. I'll be adding to the list
below throughout the semester.
**Useful Links**
. [Legacy OpenGL Red Book](https://w2.mat.ucsb.edu/594cm/2010/docs/RedBook.pdf). Reference for the fixed-function legacy OpenGL API
. [OpenGL on-line documentation](https://www.opengl.org/sdk/docs/). Reference for the OpenGL API.
. [GLSL documentation](https://www.opengl.org/documentation/glsl/). Reference for GLSL shader code.
. [WebGL Fundamentals](https://webglfundamentals.org/webgl/lessons/webgl-fundamentals.html). Rich resource on WebGl programming.
. [GL matrix documentation](https://glmatrix.net/). Documentation for a Javascript matrix/vector library.
. [Eloquent Javascript](https://eloquentjavascript.net/). A free textbook on Javascript programming.
. [Node.js](https://nodejs.org/en/docs/). A javascript interpreter that runs in terminal.
. [The Book of Shaders](https://thebookofshaders.com/). A text and an [on-line shader IDE](https://thebookofshaders.com/).
. [ShaderToy](https://www.shadertoy.com/). People's shared shader code.
......................... . . . . . . . . . . . . . . . .